Ok, this might be a bit of a challenge but fortunately the most excellent Jonathan Cauldwell, the developer of AGD, posted a YouTube video of creating energy /time bars etc in MPAGD which I am going to shamelessly use as the basis for this tutorial.
For Stinky Dog, I want an 'Air Toxicity Meter' that measures how toxic the air in the room has become due to Stinky Dogs farting, the air purity needs to be able to both rise and fall, and I want it displayed as a meter.
To start with, we'll need a variable that measures the current level of air purity, we'll use 'P'.
To draw the meter, we're also going to need a variable that stores the y co-ordinate, we can't use 'Y' as it is reserved, we'll use Q instead.
We'll then use the PLOT command to draw a series of pixels that will make up the meter. PLOT P Q will draw a pixel at the screen co-ordinate where x = P and y = Q.
We'll then extend the routine so that it changes the P & Q values and draws the meter:
As every time we start a screen, we want the air to be 100% pure, the best place to initialise it will be the RESTART SCREEN event, drop in this code...
LET P = 0 ; initial air purity level
WHILE P < 100 ; has P reached 100 yet?
LET Q = 178 ; starting y co-ord for meter
WHILE Q < 182 ; ending y co-ord for meter
PLOT P Q ; put a dot at P Q
ADD 1 TO Q ; increment Q
ENDWHILE ; keep doing this until weve drawn 4px
ADD 1 TO P ; increment P
ENDWHILE ; keep doing this until P reaches 100
So, we start with P (the x co-ord) being 0 and Q being 178.
Why 178? Remember the y value on a spectrum screen goes from top to bottom, from 0 to 191. I want the bar to be 4 pixels high starting at y = 178
The outer WHILE...ENDWHILE loop ensures we draw a bar 100px wide, the inner END...WHILE loop ensures the bar is 4px high.
OK, that should draw the air purity meter when the screen starts.
How do we make the meter go up and down?
Let's make the meter go down (meaning the air is becoming more toxic) when Stinky Dog farts.
If you recall, in Part 16 we used a variable (F) to indicate that there was a Fart currently on the screen. So if we test if F = 1 we will know there is a Fart in play, and if so we will run some code that reduces the air purity.
We're going to put this code into our Main Loop 2, so it runs at the end of each cycle, we might move it later, but this should work...
IF F = 1 ; is there a fart in play?
SUBTRACT 1 FROM P ; if so, reduce air purity by 1
LET Q = 178 ; y co-ord start at 178
WHILE Q < 182 ; run this 4 times
PLOT P Q ; plot a pixel at P Q
ADD 1 TO Q ; increment Q (the y value)
ENDWHILE
ENDIF
We'll also add some code to the PLAYER EVENT that will kill Stinky Dog if the air becomes too toxic...
IF P <= 0 ; if air purity is 0
KILL ; kill stinky dog
ENDIF
What about increasing the meter?
I'm going to create a new OBJECT, a can of Air Freshner, if Stinky Dog gets one of these it will improve the air purity, something like this should do for now:
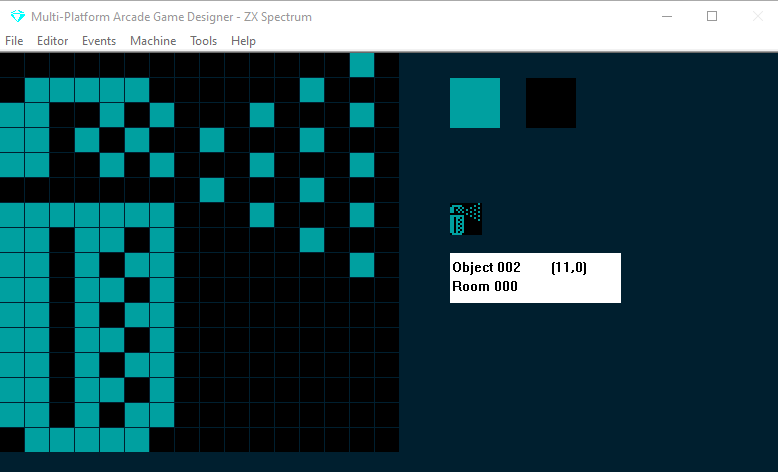
I've also put the starting position for this object in Screen 0 (click the white box to cycle through to Room 0 then hit P to go to the room, and click in the room where you want its starting position to be.
As we did in PART 17 , we're going to add some code to the Player event that controls what should happen if Stinky Dog collects this Object:
DETECTOBJ ; is sprite touching an object?
IF OBJ <> 255 ; did we detect an object?
GET OBJ ; automatically pick it up.
IF OBJ = 0 ; is it the Dog Bowl
AT 10 4 ; at line 10 column 4
COLOUR 13 ; green ink on blue paper
PRINT "you got the dog food bowl!" ; display a message
DELAY 50 ; wait a couple of seconds
WAITKEY ; then wait for a key press
REDRAW ; redraw the screen & remove the message
ENDIF
IF OBJ = 2 ; air freshner
REPEAT 20 ; do this 20 times
IF P < 100 ; prevent P from exceeding 100
LET Q = 178 ; starting y-co-ord
WHILE Q < 182 ; do this 4 times
PLOT P Q ; plot a pixel
ADD 1 TO Q ; increment the y co-ord
ENDWHILE
ADD 1 TO P ; increment the x co-ord
ENDIF
ENDREPEAT
ENDIF
ENDIF
Time for a test...
Hopefully you noticed that the meter goes down when the fart is in play and went up by 20px when we collected the air freshner.
That'll do for now, I'm not going to get hung up on the amount it rises and falls, now that we have the basic mechanics working we can always tune it later. We'll also redesign the bar to make it look better. These are just cosmetics, we've done the hard work!.
Also, when the meter fell to the left edge, Stinky Dog died.
That's a few ways we can kill him now, it's probably a good idea that we improved the death routine as right now, he just instantly respawns if we still have lives left.
Comments